React Http Request via Axios
- HeySoftware!
- Sep 8, 2018
- 3 min read
Updated: Sep 18, 2018
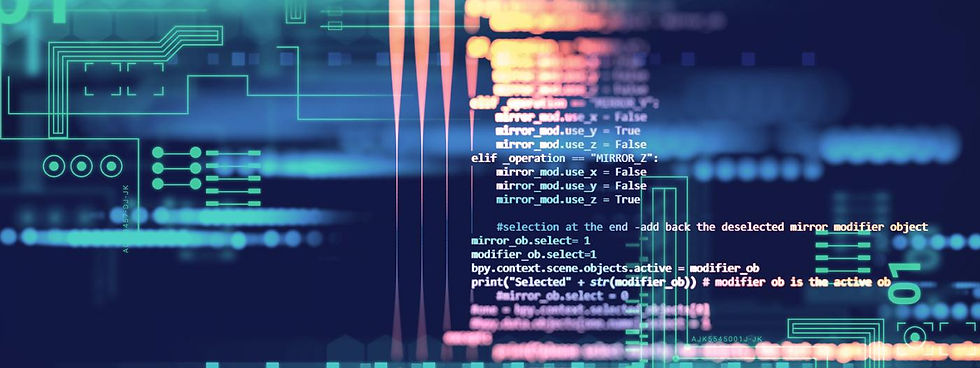
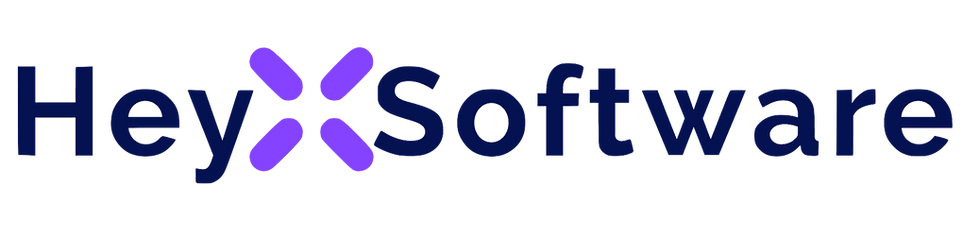
This is a quick tutorial to get you up and running with a working React application as quickly as possible. When learning a new technology there are TONS of documentation that can be overkill for getting your feet wet in an effective way. This tutorial is for people that prefer to learn by Reverse Engineering and tinkering. I will I will not not go very deep in depth of how things work, but rather give you everything you need to have a 100% functioning result. This will allow you to tear it apart and in order to learn rapidly. So lets get started!
Generate a React app
First we want to install Node by going here: https://nodejs.org/en/
Lets the latest create-react-app functionality so that we can easily generate a basic app template to work with. Go to you command terminal and type:
npm install -g create-react-app
Paste the following command into your terminal in order to generate a new React app.
create-react-app ReactHttpDemo
Start your server
cd into you newly created application and type the following command into your terminal:
npm start
Navigate to http://localhost:3000/ within your browser and you should see something similar to the image below. Good job! you have a basic React app running.
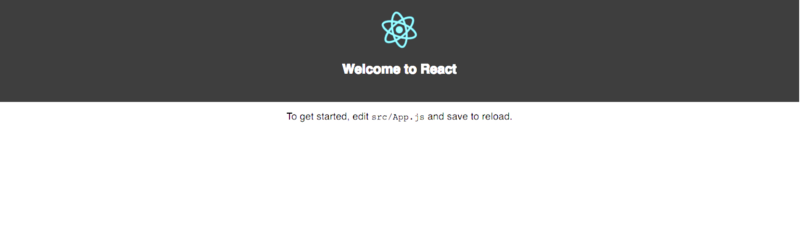
Installing Axios (Will be needed to run your code)
Run the following command within terminal to install Axios:
npm install axios -S
React is a library and not a full featured framework. We are responsible for leveraging a third party package for handling http. Axios was chosen in this example. Axios leveraged promises which might be worth studying if you don’t know about them.
Getting to the code
Under the src directory, create an new folder named components. Inside this folder create a blank JavaScript file named Item.js. Your folder structure should now look similar to the below image.
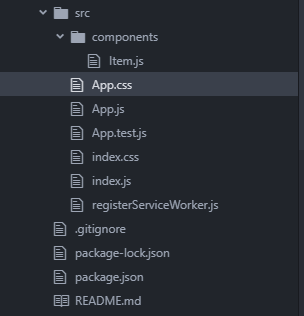
Replace the contents of the src/App.css file with:
Replace the contents of the src/App.js file with:
Replace the contents of the src/components/Item.js file with:
Lets include bootstrap into our pasting the following cdn within the head of the public/index.html file:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.0/css/bootstrap.min.css"
integrity="sha384-9gVQ4dYFwwWSjIDZnLEWnxCjeSWFphJiwGPXr1jddIhOegiu1FwO5qRGvFXOdJZ4"
crossorigin="anonymous">
Running the Result
Type the following code into your terminal in order to run your solution:
npm start
Navigate to http://localhost:3000/ within your web browser and you should see something similar to the image below:
Click the button and you should see something similar to the image below:
Understanding what happened
Your application should now be working. Your base React component is called App which is defined in the src/App.js file. It’s job is to render the purple button that is displayed in your web browser. Upon clicking this button the handle click function is called.
Handle click uses Axios to make an HTTP request to the following endpoint, https://jsonplaceholder.typicode.com/users. The response data is then stored into the component state variable to be used by the Item component. Give this endpoint a look if you are curios as to what the data looks like. Ounce the component state contains data for persons state variable, it is then passed as a property to the Item component.
The data is used to populate the Item component with data that it needs to render.
Thats it!
This tutorial is meant to kick start the process of learning React. The goal was to get you to a working app as fast as possible. This way we can do what we do best…. Reverse Engineer!!!! I personally learn extremely fast this way and wanted to provide this for others who happen to appreciate a similar approach to learning.
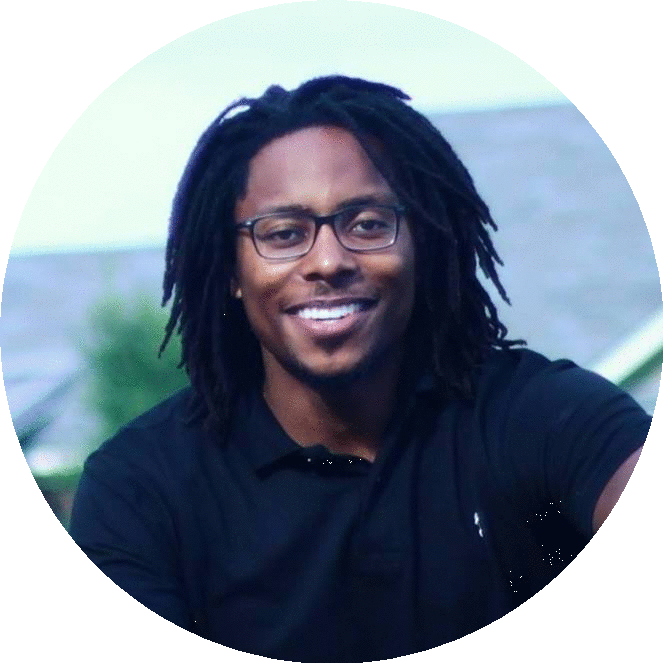
Cadarrius McGlown
Lead Software Architect
Heysoftwaresolutions.com
Comentários